Understanding Inheritance in Object-Oriented Programming (OOP)
Inheritance is one of the principles of Object-Oriented Programming Systems (OOPS).It’s about inheriting the parent properties and reusing it and helps to avoid code duplicity.
Definition: Inheritance is a mechanism in which one class acquires all the properties and behavior of another class with a specific relationship.
Why should we use it ?
Lets us take an example, Imagine you’re building a vehicle. There are certain common properties and behaviors that every vehicle must have, such as the ability to start and stop. When designing a car, you should first include those essential vehicle properties, and then you can add any unique features specific to the car, like air conditioning or a music system. This way, the car inherits the basic functionality of a vehicle while allowing for additional customization.
Technically side: In Java Programming this can be implemented using the Inheritance. The Inheritance can be implemented in multiple ways ie., multi-level inheritance, multiple inheritance.
Multi-Level inheritance, is when a class inherits from another class, and then another class inherits from that class. In simple terms, it’s like a family tree:
- The grandparent class gives common traits to the parent class.
- The parent class passes down those traits to the child class.
This allows a class to inherit features from multiple levels in the hierarchy, not just from one. For example, a Vehicle
class might pass its features to a Car
class, which then passes its features to a SportsCar
class.

Explanation:
- The
SportsCar
class inherits features from both theCar
class and theVehicle
class. - The
Vehicle
class provides thestart()
method. - The
Car
class adds adrive()
method. - The
SportsCar
class adds its ownspeedUp()
method.
This shows how the SportsCar
class can use functionality from both Vehicle
and Car
classes.
Multiple Inheritance, A class that can inherit features from more than one parent class is called multiple inheritance and the usual way to do this is by using the extends
keyword, where a class can inherit from another class.
There is a problem when we try to use the extends
keyword for multiple inheritance. Java doesn't allow a class to inherit from more than one class at a time because of the diamond problem.
The diamond problem occurs when two parent classes have the same method, and a subclass inherits from both. The subclass then doesn’t know which version of the method to use.
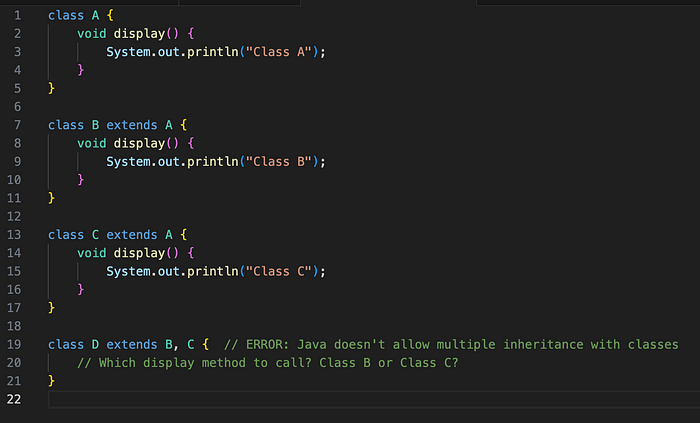
In this case, class D
inherits display()
from both B
and C
, and there’s ambiguity about which display()
method should be called.
How it’s resolved in Interfaces:
Java solves this problem using interfaces. Unlike classes, interfaces do not have implementation code, just method signatures. So, there is no conflict when a class implements multiple interfaces with the same method signatures, because the class is forced to provide its own implementation.
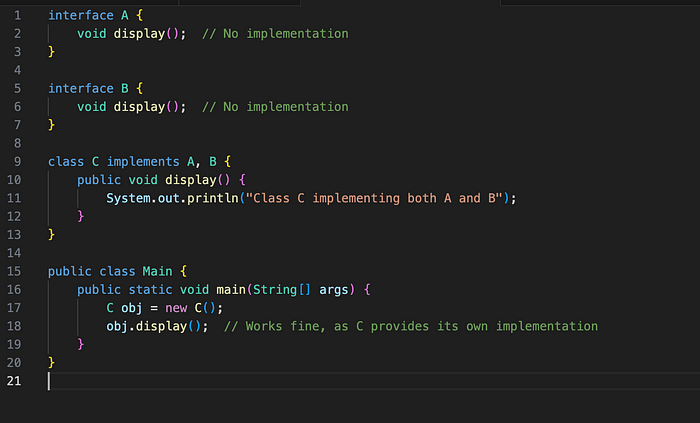
Since interfaces only define method signatures and do not provide implementations, there is no ambiguity. The class implementing multiple interfaces is responsible for providing its own implementations, thus avoiding any conflicts.
But, In Java 8, the diamond problem still exists conceptually when dealing with multiple inheritance of interfaces, but it is handled differently due to the introduction of default methods in interfaces.

Here,
- Both
A
andB
have the same default methodprint()
. - When class
C
implements bothA
andB
, it inherits bothprint()
methods. - Java cannot decide which method to call, so it gives an ambiguity error.
To resolve this, Java provides a mechanism that forces the implementing class to explicitly choose which method it wants to use, either by overriding the method or by using super to specify which parent method to call.
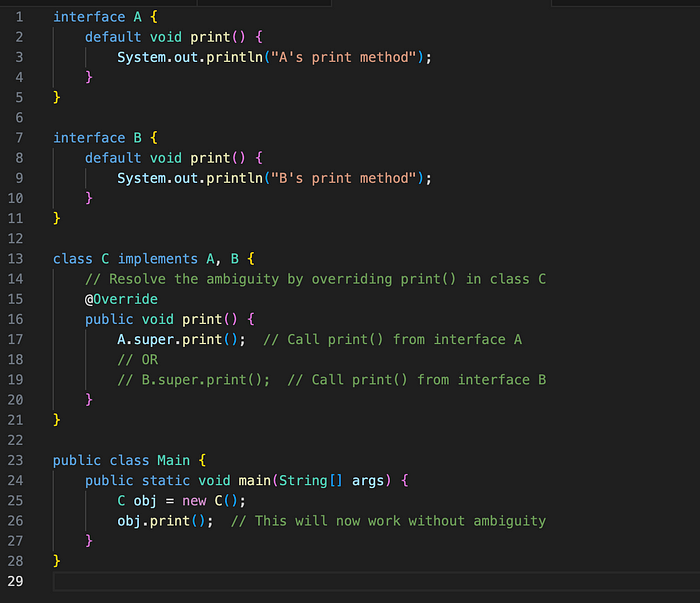
Explanation:
- In this example, class
C
overrides theprint()
method and explicitly decides which interface’s method to invoke. - The
A.super.print()
syntax allows the classC
to call the default methodprint()
from interfaceA
. Similarly, you could useB.super.print()
to call the method fromB
. - This resolves the ambiguity and avoids the diamond problem.